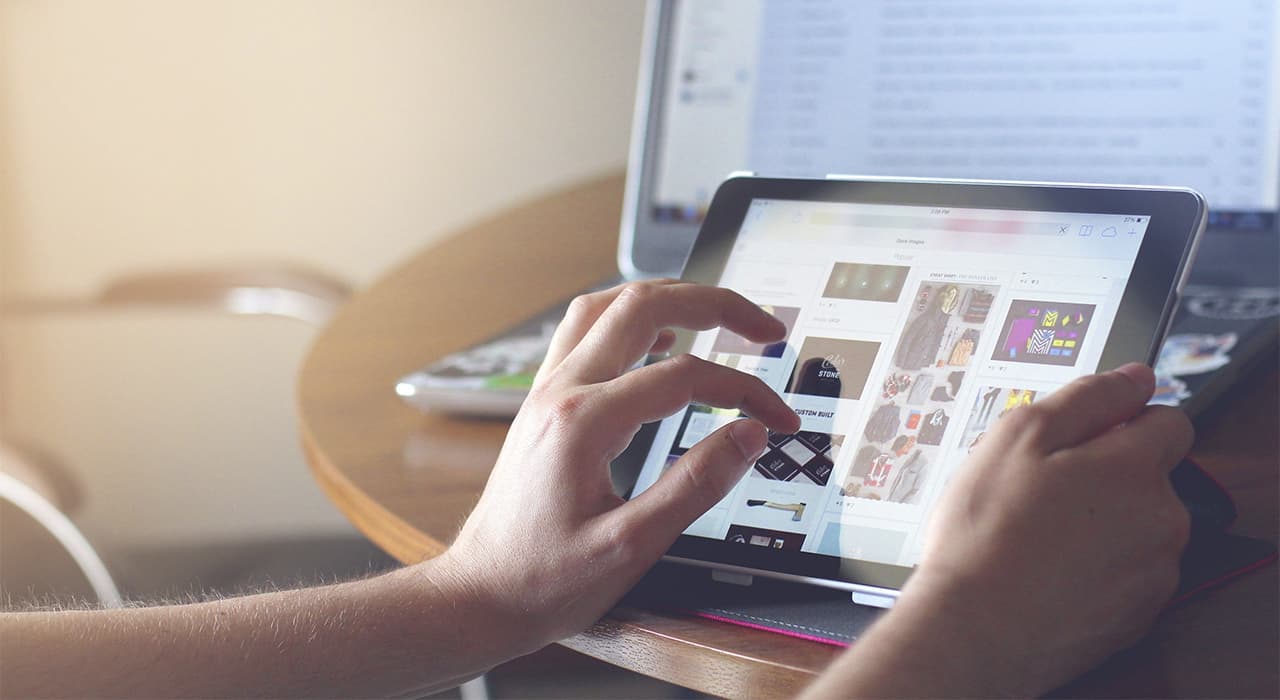
If you’re seriously considering developing your own web application, answer two questions first:
What task will this application be used for?
How will the application be developed?
- Working with the business logic of the backend
There are two ways of doing this: you can group the backend business logic in one service (monolithic logic) or implement each of its components in a separate microservice. If you work with a small project, use the first method, and if you work with a large project, the second would be ideal.
- Choosing a programming language
If you are less concerned about the web application performance, write in Python (Django, Flask frameworks), Node JS (Express JS, Koa JS, Gatsby JS frameworks), Ruby (Ruby on Rails, Grape frameworks). If speed is a priority – use Golang (Gingonic, Beego, Revel frameworks). You can also use Microsoft’s popular programming language C#, pronounced “c-sharp”. It was developed as an application layer language for the CLR. C# has absorbed much from C++, Modul, Delphi, Smalltalk, and Java, but the difference is that C# eliminates models that have proven to be problematic in SAR development. For example, C#, unlike C++, does not support multiple class inheritance, but does allow multiple implementations of interfaces. The main thing, whichever language you choose, is to code in the one you know well.
- Implementing the business logic
Focus on MVC pattern at first, and when you find that the business logic starts to get complicated, use presenter and interactor. But remember that presenter and interactor are at different levels and perform different semantic and functional loads.
Presenters handle user interface (UI) events and act as callbacks from internal layers (Interactors). Presenters are easy to test and their task is to take information from the web application and transform it to bring presenters to the screen using a view.
- QA testing the backend
Testing is a must-do so that you know if the business logic of your web application works properly, and so that you don’t have to constantly “manually” check that the code works. Use automated testing for modules and libraries, UI/UX and API compliance. Write out multiple testing options. Develop a roadmap for the platform to manage testing for all types of testing. Be sure to make sure to plug in tools to track current code coverage to make sure your web application doesn’t “hang” and runs without bugs or interruptions.
- Adding Swagger Support.
Swagger is a “smart” documentation RESTful web-API. Essentially, it’s a framework for the REST API specification, giving you the ability to not only view the specification interactively, but also send requests, called Swagger UIs. Now about the web application.
- Working with frontend business logic
The complexity of working with frontend business logic is that there are so many frameworks. Usually in modern programming we use Angular, React, Vue. They all have their advantages as well as their disadvantages. But I recommend you to choose React for your frontend work because it is lighter, simpler and more flexible.
- QA testing the frontend.
The frontend is tested by two main types of tests – logic and mapping. Logic tests verify the logical implementation of functions and classes. Display tests ensure that the content is presented to the user in the way you intended when writing the frontend. For frontend QA testing, use frameworks such as Mocha, Chai, Jest, Ava, Enzyme, Jest – they are the best-selling, easiest to use, and most understandable of all.
- Monitoring the quality of the web application
When you’ve completed step seven, your web application is, you could say, ready. Well, or it’s at the final stage of being 98% ready. What do you need to know as a result? Naturally, the first thing you need to know is how well the application is implemented, how it will work, and how long its durability will last. Lighthouse, an automated, open-source tool for monitoring the quality of your web application, can help you do just that. Lighthouse conducts system audits of web application performance and availability for the average user to understand.
The data collected with Lighthouse helps you to further tweak your web application, change details, or add and optimize new features as needed.